Game port pendant for Mach3
E-Mail me if you'd like to know more.OK, this is a very preliminary page about my experiments implementing feed rate and spindle speed overrides using the joystick interface (game port).
The game port is a very simple analog input interface that's been around for years. A good primer can be found here
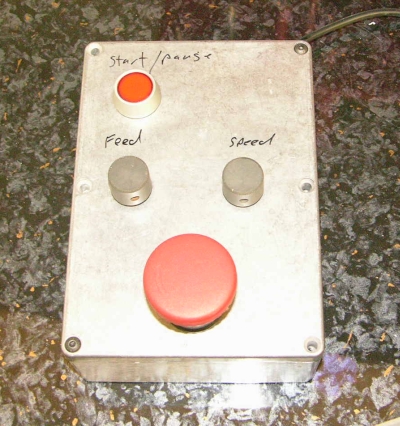
Right now I've just thrown it in this box because the monitor and keyboard console I'm building isn't done (read started) yet.
Wiring it up
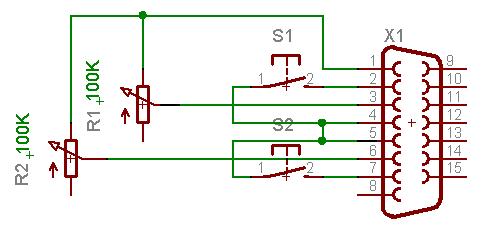
My setup is pretty simple, make sure you use linear pots though. Additional switches can be wired to pins 10 and 14 (pulling these pins to the same earth as the two swithces shown).
But I don't have a game port :-(( !
Now my machine didn't actually have a game port either, so I picked up one of these from EBay. I reckon it's actually a good way to go as it makes the whole thing plug and play. The plan is to incorporate a little USB hub so I can plug memory sticks into the console too.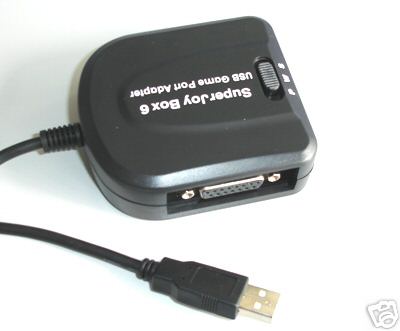
Configuring the joystick
Having plugged it in, use the game controllers applet in the windows control panel to set up the range of motion (just follow the calibration process)Configuring Mach
I first had a go at modifying the joystick plugin, got stuck when I realised that the express editions of visual C++ won't compile MFC code.So I wrote this macropump. The code to handle the buttons is a bit poxy, Bitwise operations seem to suffer from spontaneous type conversions, or something else I couldn't figure out any help with this would be appreciated.. Aside from that, it seems to work.
The two buttons are configured as E Stop, and a Start/Feedhold toggle.
Download macropump.m1s
Declare Function joyGetPos Lib "winmm.dll" (ByVal uJoyID As Long, pji As JOYINFO) As Long
Dim x As Long
Dim y As Long
Dim Buttons As Integer
Dim FRO As Single
Dim SSO As Single
Global BState As Long
Type JOYINFO
End Type
Const JOY_BUTTON1 = 1
Const JOY_BUTTON2 = 3
Const JOY_BUTTON3 = 5
Const JOY_BUTTON4 = 9
Const JOY_BUTTON5 = 17
Const JOY_BUTTON6 = 33
Const JOY_BUTTON7 = 65
Const JOY_BUTTON8 = 129
Const FeedRateOverRide = 821
Const SpindleOverRide = 74
Const bPause = 1001
Const bStart = 1000
Const bResume =1005
Const bReset = 1021
Const MaxX = 65535
Const MinX = 0
Const MaxY = 65535
Const MinY = 0
Const MidX =MinX+(MaxX-MinX)/2
Const MidY =MinY+(MaxY-MinY)/2
Dim ji As JOYINFO
' Get Joystick reading
If(joyGetPos(0, ji)=0) Then 'skip the rest if we don't see a joysick connected
End If